I’d created a couple of quick code snippets to export Microsoft Stream transcripts & someone asked if you could include a way for users to click on a hyperlink and pop into the video at the right spot for the line in the transcript they clicked. Seemed like a good idea — I’ve searched though my meeting transcript & now I want to see/hear that important part in the original video.
The method I’m using to grab the transcript text actually grabs a LOT of information that’s thrown into an object being called ‘t”:
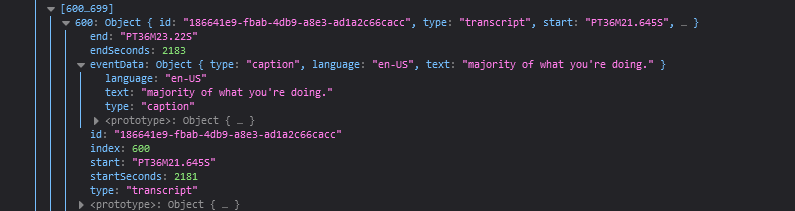
I was only using t.eventData.text to build my transcript. What do you need in order to create a jump-to-this-timecode URL for a Stream video? I had no idea! Luckily, MS supplied an easy answer — if you share a video, one of the options is to start the video at a specific time. If you pass in “st” (which I assume stands for ‘start time’) and the number of seconds ( (17 * 60) + 39 = 1059, so the 17:39 from my video matches up with 1059 seconds in the st)
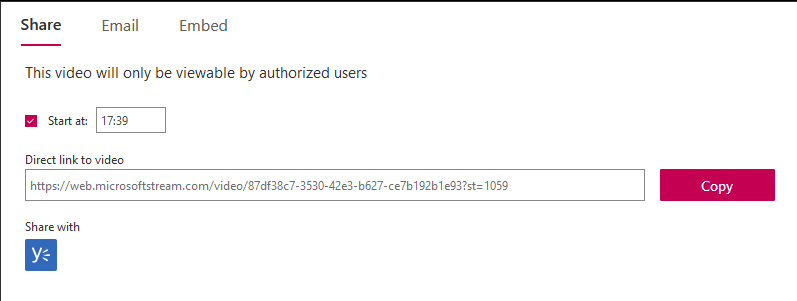
We still need the unique ID assigned to the video, but … I’m exporting the transcript from MS’s Stream site, which includes the ID in the URL. So I’m able to use window.location.href to get the URL, then strip everything past the ? … now I’ve got a way to create timecoded links to video content. I just need to glom that into the code I am using to export the transcript.
Question is … how to display it to the user? Clicking on a link for 1059 seconds doesn’t really mean anything. If I were doing this at work, I might pass the number of seconds through a “pretty time” function to convert that number of seconds back into hour:minute:second format so the user clicks on 17:39 … but, as a quick example, this builds hyperlinks with the integer number of seconds as text:
var strURL = window.location.href;
strURLBase = strURL.substring(0, strURL.indexOf('?'));
var arrayTranscriptionLines = window.angular.element(window.document.querySelectorAll('.transcript-list')).scope().$ctrl.transcriptLines.map((t) => {
var strTimecodeURL = '<a href="' + strURLBase + '?st=' + t.startSeconds + '">' + t.startSeconds + '</a>'
return strTimecodeURL + "&nbps;&nbps;&nbps;&nbps;" + t.eventData.text;
});
console.log(arrayTranscriptionLines);
I might also just link the transcript text to the appropriate URL. Then clicking on the text “I want you to remember this” would jump you to the right place in the video where that line occurs:
var strURL = window.location.href;
strURLBase = strURL.substring(0, strURL.indexOf('?'));
var arrayTranscriptionLines = window.angular.element(window.document.querySelectorAll('.transcript-list')).scope().$ctrl.transcriptLines.map((t) => {
var strResult = '<a href="' + strURLBase + '?st=' + t.startSeconds + '">' + t.eventData.text + '</a>'
return strResult;
});
console.log(arrayTranscriptionLines);
And we’ve got hyperlinked text that jumps to the right spot:
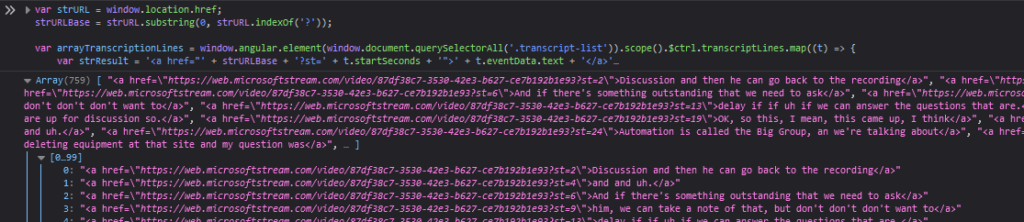